#include<stdio.h> #include<malloc.h> void insert(); void del(); void display(); struct node { int priority; int info; struct node *next; }*start, *q, *temp, *new; typedef struct node *N; int main() { int ch; do { printf( "\n[1] INSERTION\t[2] DELETION\t[3] DISPLAY [4] EXIT\t:" ); scanf( "%d", &ch ); switch ( ch ) { case 1: insert(); break; case 2: del(); break; case 3: display(); break; case 4: break; } } while ( ch < 4 ); } void insert() { int item, itprio; new = ( N* ) malloc( sizeof( N ) ); printf( "ENTER THE ELT.TO BE INSERTED :\t" ); scanf( "%d", &item ); printf( "ENTER ITS PRIORITY :\t" ); scanf( "%d", &itprio ); new->info = item; new->priority = itprio; if ( start == NULL || itprio < start->priority ) { new->next = start; start = new; } else { q = start; while ( q->next != NULL && q->next->priority <= itprio ) q = q->next; new->next = q->next; q->next = new; } } void del() { if ( start == NULL ) { printf( "\nQUEUE UNDERFLOW\n" ); } else { new = start; printf( "\nDELETED ITEM IS %d\n", new->info ); start = start->next; free( start ); } } void display() { temp = start; if ( start == NULL ) printf( "QUEUE IS EMPTY\n" ); else { printf( "QUEUE IS:\n" ); while ( temp != NULL ) { printf( "\t%d[priority=%d]", temp->info, temp->priority ); temp = temp->next; } } }
Thursday, November 24, 2011
Basic Priority queue in C
The source code of basic priority queue is given :
Thursday, November 10, 2011
Hadoop Simple Indexer source code with Map reduce
/* * To change this template, choose Tools | Templates * and open the template in the editor. */ package lineindexer; /** * * @author masteruser */ import java.io.IOException; import java.util.ArrayList; import java.util.Iterator; import java.util.List; import java.util.StringTokenizer; import org.apache.hadoop.conf.Configuration; import org.apache.hadoop.conf.Configured; import org.apache.hadoop.filecache.DistributedCache; import org.apache.hadoop.fs.Path; import org.apache.hadoop.io.LongWritable; import org.apache.hadoop.io.Text; import org.apache.hadoop.mapred.FileInputFormat; import org.apache.hadoop.mapred.FileOutputFormat; import org.apache.hadoop.mapred.FileSplit; import org.apache.hadoop.mapred.JobClient; import org.apache.hadoop.mapred.JobConf; import org.apache.hadoop.mapred.MapReduceBase; import org.apache.hadoop.mapred.Mapper; import org.apache.hadoop.mapred.OutputCollector; import org.apache.hadoop.mapred.Reducer; import org.apache.hadoop.mapred.Reporter; import org.apache.hadoop.mapred.TextInputFormat; import org.apache.hadoop.mapred.TextOutputFormat; import org.apache.hadoop.util.Tool; import org.apache.hadoop.util.ToolRunner; public class Main extends Configured implements Tool{ public static class LineIndexMapper extends MapReduceBase implements Mapper<LongWritable, Text, Text, Text> { private final static Text word = new Text(); private final static Text location = new Text(); public void map(LongWritable key, Text val, OutputCollector<Text, Text> output, Reporter reporter) throws IOException { FileSplit fileSplit = (FileSplit)reporter.getInputSplit(); String fileName = fileSplit.getPath().getName(); location.set(fileName); String line = val.toString(); StringTokenizer itr = new StringTokenizer(line.toLowerCase()); while (itr.hasMoreTokens()) { word.set(itr.nextToken()); output.collect(word, location); } } } public static class LineIndexReducer extends MapReduceBase implements Reducer<Text, Text, Text, Text> { public void reduce(Text key, Iterator<Text> values, OutputCollector<Text, Text> output, Reporter reporter) throws IOException { boolean first = true; StringBuilder toReturn = new StringBuilder(); while (values.hasNext()){ if (!first) toReturn.append(", "); first=false; toReturn.append(values.next().toString()); } output.collect(key, new Text(toReturn.toString())); } } /** * The actual main() method for our program; this is the * "driver" for the MapReduce job. */ public int run(String[] args) throws Exception { JobConf conf = new JobConf(getConf(), Main.class); conf.setJobName("LineIndexer"); conf.setOutputKeyClass(Text.class); conf.setOutputValueClass(Text.class); conf.setMapperClass(LineIndexMapper.class); conf.setReducerClass(LineIndexReducer.class); conf.setInputFormat(TextInputFormat.class); conf.setOutputFormat(TextOutputFormat.class); int i=args.length; FileInputFormat.setInputPaths(conf, new Path(args[i-2])); FileOutputFormat.setOutputPath(conf, new Path(args[i-1])); JobClient.runJob(conf); return 0; } public static void main(String[] args) throws Exception { int res = ToolRunner.run(new Configuration(), new Main(), args); System.exit(res); } }
Tuesday, November 1, 2011
SCTP server client Code in C
Client.c
#include <sys/socket.h> #include <sys/types.h> #include <netinet/in.h> #include <netinet/sctp.h> #include <arpa/inet.h> #include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <errno.h> #define RECVBUFSIZE 4096 #define PPID 1234 int main() { int SctpScocket, in, flags; socklen_t opt_len; char * szAddress; int iPort; char * szMsg; int iMsgSize; char a[1024]; struct sockaddr_in servaddr = {0}; struct sctp_status status = {0}; struct sctp_sndrcvinfo sndrcvinfo = {0}; struct sctp_event_subscribe events = {0}; struct sctp_initmsg initmsg = {0}; char * szRecvBuffer[RECVBUFSIZE + 1] = {0}; socklen_t from_len = (socklen_t) sizeof(struct sockaddr_in); //get the arguments szAddress = "127.0.0.1"; iPort = 5001; printf("Starting SCTP client connection to %s:%u\n", szAddress, iPort); SctpScocket = socket(AF_INET, SOCK_STREAM, IPPROTO_SCTP); printf("socket created...\n"); //set the association options initmsg.sinit_num_ostreams = 1; setsockopt( SctpScocket, IPPROTO_SCTP, SCTP_INITMSG, &initmsg,sizeof(initmsg)); printf("setsockopt succeeded...\n"); bzero( (void *)&servaddr, sizeof(servaddr) ); servaddr.sin_family = AF_INET; servaddr.sin_port = htons(iPort); servaddr.sin_addr.s_addr = inet_addr( szAddress ); connect( SctpScocket, (struct sockaddr *)&servaddr, sizeof(servaddr)); printf("connect succeeded...\n"); //check status opt_len = (socklen_t) sizeof(struct sctp_status); getsockopt(SctpScocket, IPPROTO_SCTP, SCTP_STATUS, &status, &opt_len); while(1) { printf("Sending Role to server: "); gets(a); sctp_sendmsg(SctpScocket, (const void *)a, iMsgSize, NULL, 0,htonl(PPID), 0, 0 , 0, 0); //read response from test server in = sctp_recvmsg(SctpScocket, (void*)szRecvBuffer, RECVBUFSIZE,(struct sockaddr *)&servaddr, &from_len, &sndrcvinfo, &flags); if (in > 0 && in < RECVBUFSIZE - 1) { szRecvBuffer[in] = 0; printf("Received from server: %s\n",szRecvBuffer); } } printf("exiting...\n"); close(SctpScocket); return 0; }
Server.c
#include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <netinet/sctp.h> #include <arpa/inet.h> #include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <errno.h> #define RECVBUFSIZE 4096 #define PPID 1234 int main() { int SctpScocket, n, flags; socklen_t from_len; struct sockaddr_in addr = {0}; struct sctp_sndrcvinfo sinfo = {0}; struct sctp_event_subscribe event = {0}; char pRecvBuffer[RECVBUFSIZE + 1] = {0}; char * szAddress; int iPort; char * szMsg; int iMsgSize; //get the arguments szAddress = "127.0.0.1"; iPort = 5001; szMsg = "Hello Client"; iMsgSize = strlen(szMsg); if (iMsgSize > 1024) { printf("Message is too big for this test\n"); return 0; } //here we may fail if sctp is not supported SctpScocket = socket(AF_INET, SOCK_SEQPACKET, IPPROTO_SCTP); printf("socket created...\n"); //make sure we receive MSG_NOTIFICATION setsockopt(SctpScocket, IPPROTO_SCTP, SCTP_EVENTS, &event,sizeof(struct sctp_event_subscribe)); printf("setsockopt succeeded...\n"); addr.sin_family = AF_INET; addr.sin_port = htons(iPort); addr.sin_addr.s_addr = inet_addr(szAddress); //bind to specific server address and port bind(SctpScocket, (struct sockaddr *)&addr, sizeof(struct sockaddr_in)); printf("bind succeeded...\n"); //wait for connections listen(SctpScocket, 1); printf("listen succeeded...\n"); int count = 0,i; char a[20][10],d[20][10]; char send_data[1024]; strcpy(a[0],"Abraham"); strcpy(a[1],"Buck"); strcpy(a[2],"Casper"); strcpy(a[3],"Dammy"); strcpy(d[0],"1"); strcpy(d[1],"2"); strcpy(d[2],"3"); strcpy(d[3],"4"); while(1) { //each time erase the stuff flags = 0; memset((void *)&addr, 0, sizeof(struct sockaddr_in)); from_len = (socklen_t)sizeof(struct sockaddr_in); memset((void *)&sinfo, 0, sizeof(struct sctp_sndrcvinfo)); n = sctp_recvmsg(SctpScocket, (void*)pRecvBuffer, RECVBUFSIZE,(struct sockaddr *)&addr, &from_len, &sinfo, &flags); if (-1 == n) { printf("Error with sctp_recvmsg: -1... waiting\n"); printf("errno: %d\n", errno); perror("Description: "); sleep(1); continue; } if (flags & MSG_NOTIFICATION) { printf("Notification received!\n"); printf("From %s:%u\n", inet_ntoa(addr.sin_addr), ntohs(addr.sin_port)); } else { printf("Received from %s:%u on stream %d, PPID %d.: %s\n", inet_ntoa(addr.sin_addr), ntohs(addr.sin_port), sinfo.sinfo_stream, ntohl(sinfo.sinfo_ppid), pRecvBuffer ); int p = 0; for(i=0;i<4;i++) { if(strcmp(pRecvBuffer,d[i]) == 0) { strcpy(send_data,a[i]);p=1; } } if(p == 0) strcpy(send_data,"No one on that role."); } //send message to client printf("Sending to client: %s\n", send_data); sctp_sendmsg(SctpScocket, (const void *)send_data, strlen(send_data), (struct sockaddr *)&addr, from_len, htonl(PPID), 0, 0 /*stream 0*/, 0, 0); //close server when exit is received if (0 == strcmp(pRecvBuffer, "exit")) { break; } }//while printf("exiting...\n"); close(SctpScocket); return (0); }To run this you have to have sctp library installed. then comand like >>$ gcc -g -lsctp filename
Subscribe to:
Posts (Atom)
How to enable hotspot in TPG iPhone
By default, the hotspot does not work on the phone. It will ask you to contact the provider. This video will help you bypass the network ...
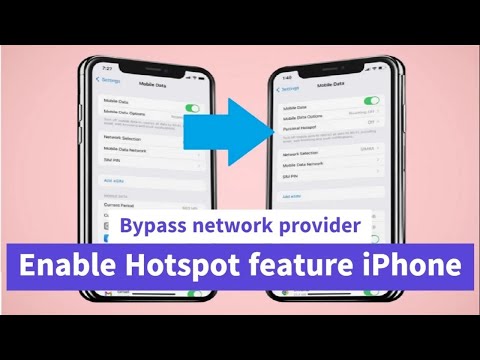
-
Server #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <arpa/inet.h> #include <...
-
There is none who can replace him.At least the standard which he create in is life time in the running literature it never be replaceable.Th...
-
ISSUE: Build error on Asterik , when you want test webrtc feature :) checking for uuid_generate_random in -luuid... no checking for uuid...